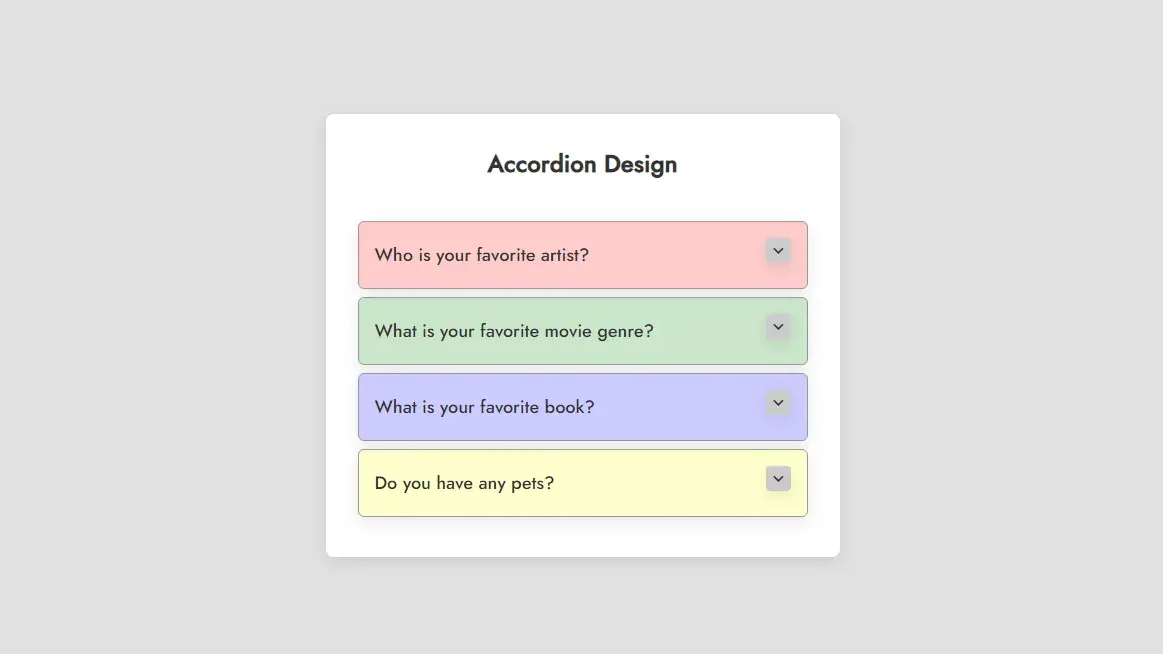
Table of Contents
Introduction: Simplest Accordion using HTML CSS JavaScript
Understanding the fundamentals of front-end development involves mastering essential components like HTML, CSS, and JavaScript. A simple accordion design is an excellent starting point. This project offers insights into manipulating the DOM, working with CSS for styling, and implementing JavaScript for interactivity.
Let’s delve into building a Simplest Accordion using HTML CSS JavaScript. The accordion consists of collapsible sections with questions and answers, providing a clean and interactive UI experience.
Steps to Set Up the Simple Accordion:
Please follow this Step-by-Step Guide to Create and Setup the Simplest Accordion using HTML CSS JavaScript —
Begin by creating a new folder for the project. Inside this folder, create and set up three files: index.html
, style.css
, and script.js
. Ensure the filenames and extensions match for consistency.
Step 1: HTML Structure
In the index.html
file, pest the provided HTML code to set up the structure of this project. This includes an overarching container for the accordion items and the individual sections containing questions and answers.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>simple Accordion using html css js</title> </head> <body> <link href='https://unpkg.com/boxicons@2.1.1/css/boxicons.min.css' rel='stylesheet'> <div class="accordion-container"> <h2>Accordion Design</h2> <div class="accordion-item"> <header class="item-header"> <h4 class="item-question"> Who is your favorite artist? </h4> <div class="item-icon"> <i class='bx bx-chevron-down'></i> </div> </header> <div class="item-content"> <p class="item-answer"> My favorite artist is Leonardo da Vinci. </p> </div> </div> <div class="accordion-item"> <header class="item-header"> <h4 class="item-question"> What is your favorite movie genre? </h4> <div class="item-icon"> <i class='bx bx-chevron-down'></i> </div> </header> <div class="item-content"> <p class="item-answer"> I enjoy watching science fiction movies. </p> </div> </div> <div class="accordion-item"> <header class="item-header"> <h4 class="item-question"> What is your favorite book? </h4> <div class="item-icon"> <i class='bx bx-chevron-down'></i> </div> </header> <div class="item-content"> <p class="item-answer"> One of my favorite books is "To Kill a Mockingbird" by Harper Lee. </p> </div> </div> <div class="accordion-item"> <header class="item-header"> <h4 class="item-question"> Do you have any pets? </h4> <div class="item-icon"> <i class='bx bx-chevron-down'></i> </div> </header> <div class="item-content"> <p class="item-answer"> Yes, I have a pet dog named Max. </p> </div> </div> </div> </body> </html>
Step 2: Styling with CSS
Now, Copy the below CSS code and paste it into your style.css
file to give your accordion a visually enhanced look. Utilize CSS properties to define the layout, including borders, colours, and transitions. The CSS also includes animations for a smooth accordion experience.
@import url("https://fonts.googleapis.com/css2?family=Jost:wght@500&display=swap"); :root { --main-bg: #e1e1e1; --border-color: #999; --box-shadow: 0px 4px 16px rgba(0, 0, 0, 0.1); --icon-bg: #ccc; } body { font-family: 'Jost', sans-serif; background: var(--main-bg); color: #333; display: grid; place-items: center; min-height: 100vh; } h2 { text-align: center; margin: 0px 0px 40px 0px; } .accordion-container { max-width: 450px; width: 100%; margin: 0 auto; padding: 2rem; background: #fff; box-shadow: var(--box-shadow); border-radius: 8px; } .accordion-item { display: flex; flex-direction: column; padding: 0 1rem; border-radius: 6px; border: 1px solid var(--border-color); box-shadow: var(--box-shadow); background: var(--main-bg); margin-bottom: 0.5em; } .accordion-item:nth-child(2) { background-color: rgba(255, 0, 0, 0.2); } .accordion-item:nth-child(3) { background-color: rgba(0, 128, 0, 0.2); } .accordion-item:nth-child(4) { background-color: rgba(0, 0, 255, 0.2); } .accordion-item:nth-child(5) { background-color: rgba(255, 255, 0, 0.2); } .item-header { display: flex; justify-content: space-between; column-gap: 0.2em; font-size: 18px; } .item-icon { margin-top: 1rem; flex: 0 0 25px; display: grid; place-items: center; font-size: 1.35rem; height: 25px; width: 25px; border-radius: 4px; background: var(--icon-bg); cursor: pointer; box-shadow: var(--box-shadow); } .item-icon i { transition: all 0.25s cubic-bezier(0.5, 0, 0.1, 1); } .item-question { font-size: 1em; line-height: 1; font-weight: 500; } .active .item-icon i { transform: rotate(180deg); } .active .item-question { font-weight: 500; } .item-content { max-height: 0; overflow: hidden; transition: all 300ms ease; } .item-answer { line-height: 150%; opacity: 0.8; }
Step 3: JavaScript Functionality
Incorporate the JavaScript code from the script.js
file. Use event listeners to toggle the active state and manage the expansion and collapse of accordion items. This code snippet utilizes a click event to expand or collapse the content.
const accordionBtns = document.querySelectorAll(".item-header"); accordionBtns.forEach((accordion) => { accordion.onclick = function () { this.classList.toggle("active"); let content = this.nextElementSibling; if (content.style.maxHeight) { content.style.maxHeight = null; } else { content.style.maxHeight = content.scrollHeight + "px"; } }; });
Conclusion:
Congratulations! You’ve crafted a Simplest Accordion using HTML CSS JavaScript. This interactive element provides a user-friendly way to display information. Feel free to customize the styles or add more features to suit your project needs.
By following these steps, you’ve not only built an accordion but also gained insight into combining these languages to create interactive components. Keep exploring and experimenting to enhance your coding prowess!
Remember, coding is a journey of exploration and creation. Practice regularly and enjoy the process of bringing ideas to life through code.
For your convenience, the total source code of this “Simplest Accordion using HTML CSS JavaScript” project instructional practice is accessible for download by clicking the Download Code button.
Note: Keep in mind that the way to dominate coding is practice. To enhance your skills in JavaScript, HTML & CSS, we recommend recreating other useful website elements such as Custom Button, Reviews Card, Contact Page, Navigation, Login Forms, etc.