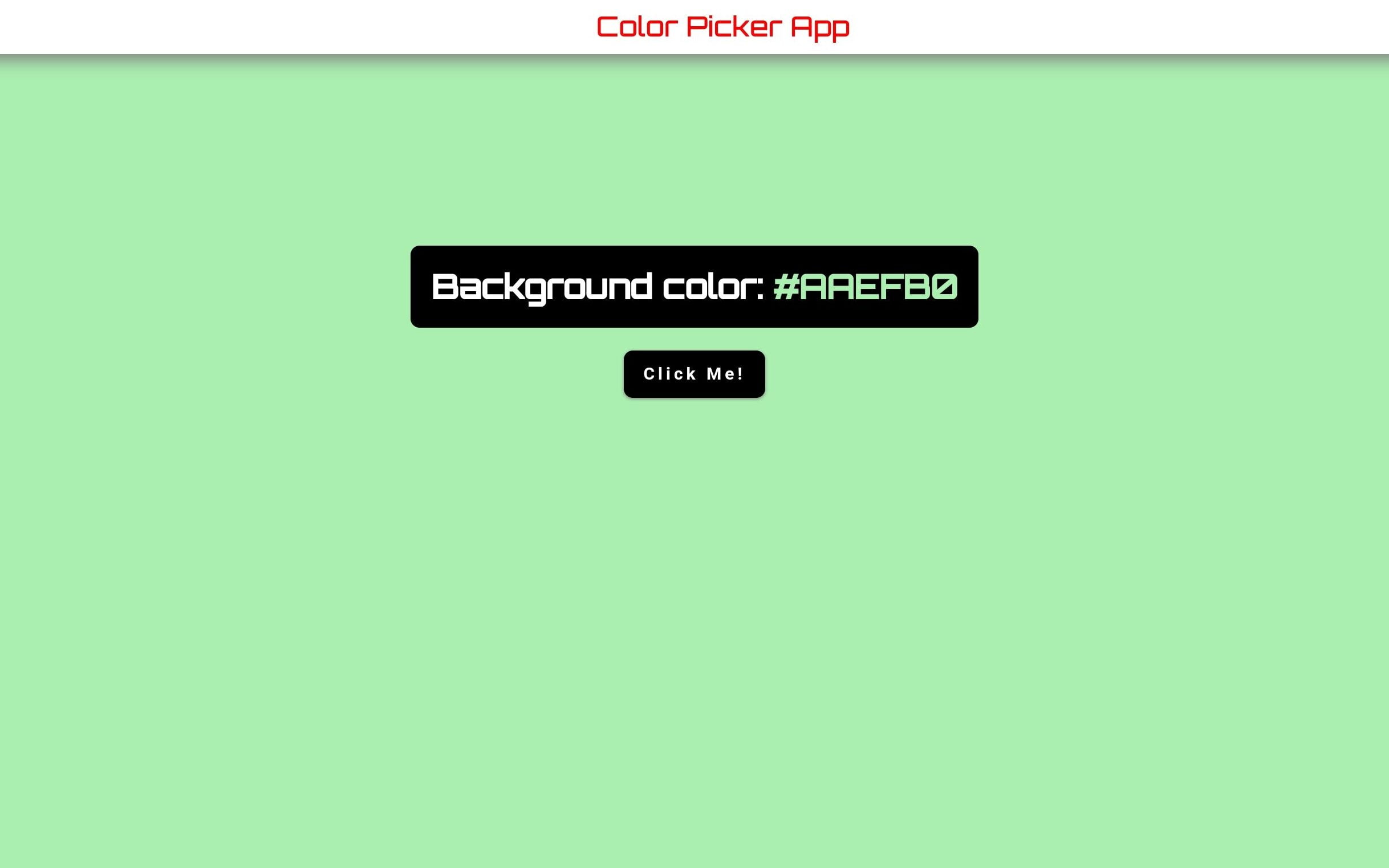
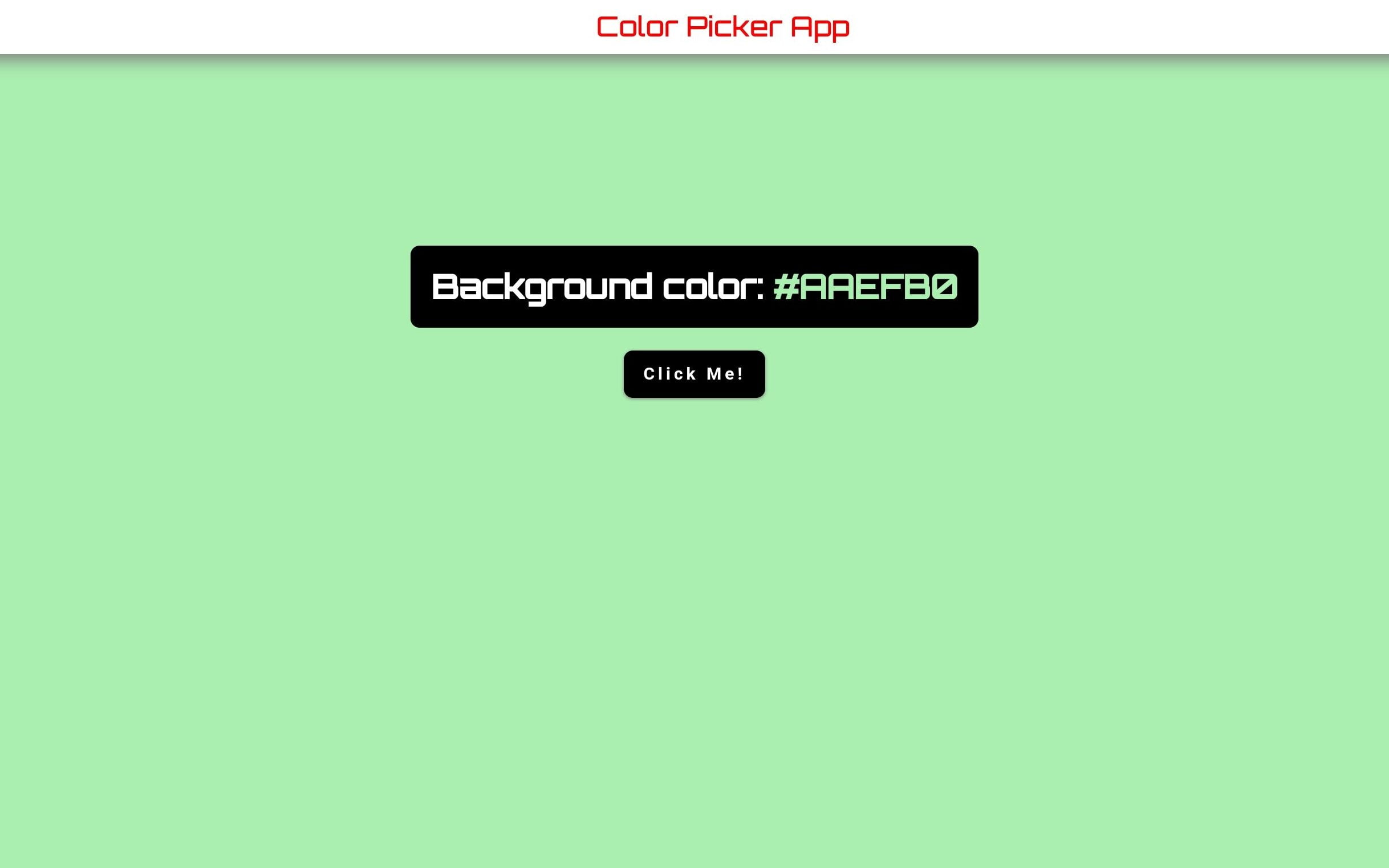
Table of Contents
Introduction: For Color Picker Tool in Javascript
Embarking on the creation of a color picker tool in Javascript, HTML and CSS is a stimulating journey that not only broadens your coding prowess but also immerses you in pivotal concepts of web development. This guide serves as a gateway for enthusiasts to craft a practical and interactive tool for color selection in their projects.
By diving into this project, you’ll venture into the intricate world of DOM manipulation, event handling, and UI design, crucial pillars in the realm of web development. Understanding these concepts will enable you to create a seamless user experience while honing your coding skills.
The color picker’s functionality lies in its ability to generate random colors on a single click, by clicking the “Click Me!” button, showcasing the hex color code for each. This engaging and educational process not only enhances your coding finesse but also equips you with a practical tool for future projects.
4 Steps to Create a Color Picker Tool in JavaScript:
If you want to create a Random Color Picker Tool in JavaScript, HTML & CSS then follow these step-by-step guide –
Step 1: Setting Up the Project:
Begin by creating a new folder for the project. Within this folder, initiate three essential files: index.html
, style.css
, and script.js
. These files are pivotal in establishing the foundation for the color picking tool. The HTML file structures the layout, the CSS file styles it, while the JavaScript file fuels functionality.
Step 2: Constructing the HTML Structure:
In the index.html
file, input the provided HTML code snippet. This code establishes the foundational layout of our color picking tool. It includes a header, a section displaying the current background color, and a button triggering the color change functionality.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Color Picker App</title> </head> <body> <nav> <div class="nav-container"> <h4>Color Picker App</h4> </div> </nav> <main> <div class="content-container"> <h2>Background color: <span class="color-span">Sky Blue</span></h2> <button class="btn btn-hero" id="btn">Click Me!</button> </div> </main> </body> </html>
Step 3: Styling with CSS:
Move on to the style.css
file and insert the provided CSS code. These style directives will enhance the visual appeal of the color picking tool, creating an engaging user interface. Feel free to experiment with the styles to suit your preferences.
@import url('https://fonts.googleapis.com/css2?family=Lato:ital,wght@0,300;0,400;1,700&family=Orbitron:wght@500&family=Oswald&family=Signika&family=Ubuntu&display=swap'); body { background-color: #f1f5f8; font-size: larger; font-family: 'Orbitron', sans-serif; margin: 0; font-family: 'Orbitron', sans-serif; } nav { background-color: #fff; box-shadow: 0 5px 15px gray; margin-bottom: 50px; height: 50px; display: flex; align-items: center; justify-content: center; } .nav-container { margin-left: 50px; } .nav-container h4 { font-size: 24px; font-weight: 500; color: #ff0000; margin: 0; } main { display: grid; place-items: center; margin-top: 10%; } .content-container{ display: grid; place-items: center; } .content-container h2 { background-color: #000; color: #fff; padding: 18px; border-radius: 8px; margin-bottom: 20px; } .color-span { color: #00bfff; } .btn-hero { text-transform: capitalize; background: transparent; color: black; letter-spacing: 3px; display: inline-block; font-weight: 700; border: 2px solid black; cursor: pointer; box-shadow: 0 1px 3px gray; border-radius: 8px; font-size: 15px; padding: 10px 15px; transition: background 1s; } .btn-hero:hover { color: #fff; background: #000; }
Step 4: Implementing JavaScript Functionality:
Within the script.js
file, copy and paste the JavaScript code provided. This code segment powers the tool’s core functionality. When the button is clicked, it generates a random hex color, applies it as the background color, and displays the corresponding hex code.
const hex = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, "A", "B", "C", "D", "E", "F"]; const btnElement = document.getElementById("btn"); const colorSpanElement = document.querySelector(".color-span"); btnElement.addEventListener("click", function () { let hexColor = "#"; for (let i = 0; i < 6; i++) { hexColor += hex[generateRandomNumber()]; } document.body.style.backgroundColor = hexColor; colorSpanElement.textContent = hexColor; colorSpanElement.style.color = hexColor; }); function generateRandomNumber() { return Math.floor(Math.random() * hex.length); }
Conclusion:
Congratulations! You’ve successfully crafted a vibrant Color Picker Tool in Javascript, HTML and CSS. This project not only hones your coding skills but also provides a practical application of web development concepts.
Remember, coding is not just about creating functional projects; it’s about exploring, experimenting, and embracing the joy of building something from scratch. Happy coding!
For your benefit, the total source code of this instructional exercise is accessible for download by clicking the Download Code button. Moreover, experience a live exhibit of these Color Picker Tool in JavaScript by getting to the View Live button.
Note: Keep in mind that the way to dominate coding is practice. To enhance your skills in HTML & CSS, we recommend recreating other useful website elements such as Card Design, Registration Forms, Navigation, Login Forms, etc.