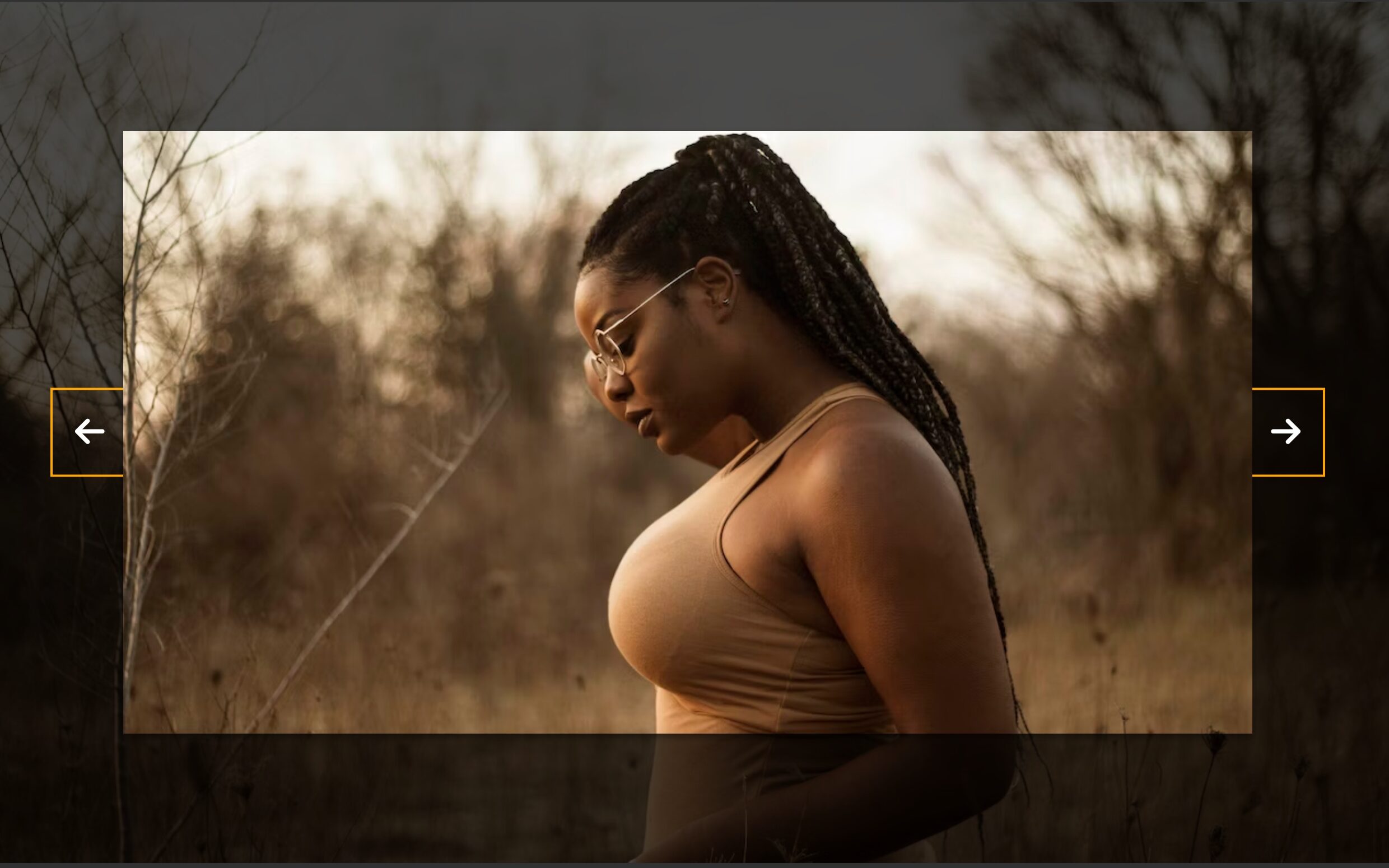
Table of Contents
Introduction: Image Slides in HTML & CSS using JavaScript
We are about to enter the dynamic web element world! Today we’re going to embark upon a fantastic journey of creating an eye-catching Image Slides in HTML & CSS using JavaScript. In addition to enhancing the looks of a web page, image sliders serve as an added value for any website and increase visitors’ interest in it.
We’ll discuss the process of creating a stunning display carousel, that complements your web projects both visually appealing as well as functionally, with this step-by-step guide. You will learn how to create a seamless and engaging slide show by the end of this session that elegantly displays your images.
3 Steps to Create an Image Slides in HTML & CSS with Javascript:
Please follow these steps to Create a Responsive Image Slides in HTML & CSS using Javascript –
Step 1: Set Up the HTML Structure:
Let’s start by organizing our project for a moment. Create a new folder, name it whatever you like, and create the index.html
, style.css
and script.js
files inside that folder. Check that the required Font Awesome Library and Google Fonts have been downloaded, as described in this code.
Now, Open the index.html
file, and input the HTML code that is given here. This will also include the structure of the document, it’s necessary XML tags, and essential links to other stylesheets.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.5.1/css/all.min.css" integrity="sha512-DTOQO9RWCH3ppGqcWaEA1BIZOC6xxalwEsw9c2QQeAIftl+Vegovlnee1c9QX4TctnWMn13TZye+giMm8e2LwA==" crossorigin="anonymous" referrerpolicy="no-referrer" /> <title>Image Slider</title> </head> <body> <div class="slider-frame"> <div class="slider-item active" style=" background-image: url('https://images.unsplash.com/photo-1702208338334-c1f15ea7f57c?q=80&w=1470&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D'); "></div> <div class="slider-item" style=" background-image: url('https://images.unsplash.com/photo-1523673671576-35ff54e94bae?q=80&w=1470&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D'); "></div> <div class="slider-item" style=" background-image: url('https://images.unsplash.com/photo-1544441543-513a50c268f1?q=80&w=1470&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D'); "></div> <div class="slider-item" style=" background-image: url('https://images.unsplash.com/photo-1525337132786-5828a43893af?q=80&w=1476&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D'); "></div> <div class="slider-item" style=" background-image: url('https://images.unsplash.com/photo-1473700216830-7e08d47f858e?q=80&w=1470&auto=format&fit=crop&ixlib=rb-4.0.3&ixid=M3wxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8fA%3D%3D'); "></div> <button class="nav-button left-nav" id="left"> <i class="fas fa-arrow-left"></i> </button> <button class="nav-button right-nav" id="right"> <i class="fas fa-arrow-right"></i> </button> </div> </body> </html>
Step 2: Style Your Image Slider:
You’ll be switching to a style.css
file. It’s where the magic appears. The CSS code is set up to define the general layout, formatting, and transitions of our image slider. Details, such as frame sizes, the opacity of items, and navigation button style should be taken into account.
/* Importing Google font - Poppins */ @import url('https://fonts.googleapis.com/css2?family=Poppins:wght@200;300;400;500;600;700;800;900&display=swap'); * { box-sizing: border-box; } body { font-family: "Roboto", sans-serif; display: flex; flex-direction: column; align-items: center; justify-content: center; height: 100vh; overflow: hidden; margin: 0; background-position: center center; background-size: cover; transition: 0.4s ease; } body::before { content: ""; position: absolute; top: 0; left: 0; width: 100%; height: 100vh; background-color: rgba(0, 0, 0, 0.7); z-index: -1; } .slider-frame { box-shadow: 0 3px 6px rgba(0, 0, 0, 0.16), 0 3px 6px rgba(0, 0, 0, 0.23); height: 70vh; width: 70vw; position: relative; overflow: hidden; } .slider-item { opacity: 0; height: 100vh; width: 100vw; background-position: center center; background-size: cover; position: absolute; top: -15vh; left: -15vw; transition: 0.4s ease; z-index: 1; } .slider-item.active { opacity: 1; } .nav-button { position: fixed; background-color: transparent; padding: 20px; font-size: 30px; color: #fff; border: 2px solid orange; top: 50%; transform: translateY(-50%); cursor: pointer; } .nav-button:focus { outline: 0; } .left-nav { left: calc(15vw - 65px); } .right-nav { right: calc(15vw - 65px); }
To add styles that supplement the subject of your site, go ahead and do so. To make it your own, you’ll need to change the textual style, change the tone, or investigate with different properties.
Step 3: Implement JavaScript for Interactivity:
At the end of the body element, we inserted a simple JavaScript script to ensure that the slider functions properly. If you click buttons, this script will guide the navigation between slides. Notice that the active slide variable is tracking the current slide as you’re reading this code.
<script> const body = document.body; const slides = document.querySelectorAll(".slider-item"); const leftButton = document.getElementById("left"); const rightButton = document.getElementById("right"); let activeSlide = 0; const setBackground = () => { body.style.backgroundImage = slides[activeSlide].style.backgroundImage; }; const setActiveSlide = () => { slides.forEach((slide) => slide.classList.remove("active")); slides[activeSlide].classList.add("active"); }; rightButton.addEventListener("click", () => { activeSlide++; if (activeSlide > slides.length - 1) activeSlide = 0; setBackground(); setActiveSlide(); }); leftButton.addEventListener("click", () => { activeSlide--; if (activeSlide < 0) activeSlide = slides.length - 1; setBackground(); setActiveSlide(); }); setBackground(); </script>
You should see a nice image slider, now that you’ve opened index.html in your web browser. To switch between images easily, press the navigation buttons.
Conclusion:
Congratulations on your mastery of creating Image Slides in HTML & CSS using JavaScript. You’ll be able to improve your website projects and appeal to the audience through striking visual content thanks to this newfound skill.
As you proceed with web development, explore the more advanced features and experiment with a variety of designs if you want to further enhance your creation. Let us remember, the Web Development Industry is full of possibilities and your expertise in creating image sliders has only just begun.
Join Our Telegram Channel to Download the Project Source Code: Click Here
For your convenience, the total source code of this “login and signup form” project instructional practice is accessible for download by clicking the Download Code button.
Note: Keep in mind that the way to dominate coding is practice. To enhance your skills in JavaScript, HTML & CSS, we recommend recreating other useful website elements such as Custom Button, Reviews Card, Contact Page, Navigation, Login Forms, etc.
More Categories:
Blog • Cards • Fun Project • Game • Hamburger Menu • Login / Signup • Navbar • Testimony / Reviews • To-Do List