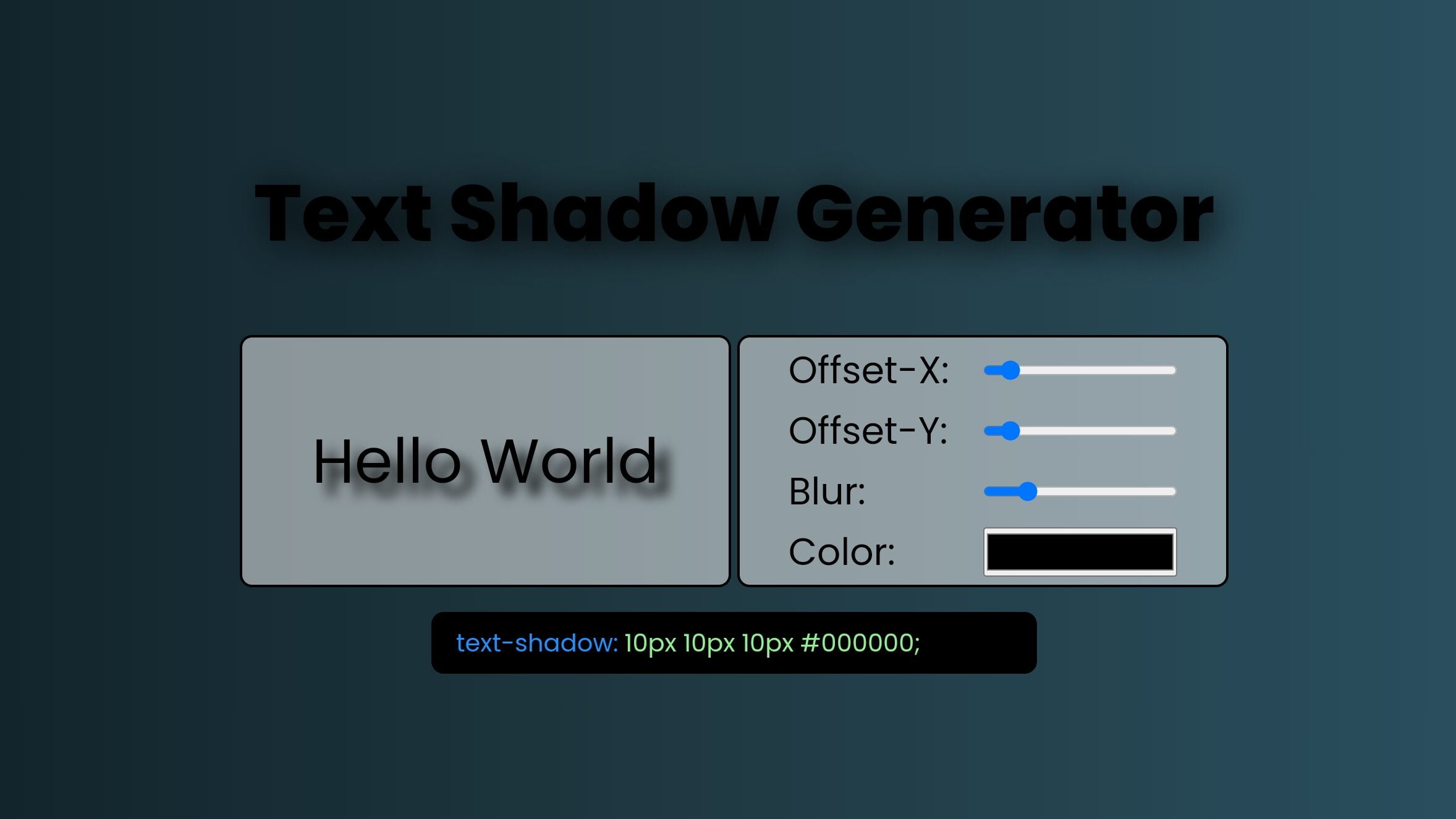
Table of Contents
Introduction:
Embarking on the journey of creating a Text Shadow Generator using HTML, CSS, and JavaScript is a fantastic way to enhance your coding skills. This project not only allows you to delve into the intricacies of manipulating styles with CSS but also provides hands-on experience with JavaScript for dynamic user interactions.
In this article, we’ll guide you through the process of setting up your very own Text Shadow Generator. By the end of this tutorial, you’ll have a responsive web application that lets you play with text shadow effects effortlessly.
Steps to Create Text Shadow Generator using JavaScript:
Please follow this Step-by-Step Guide to Create and Setup the Simplest Accordion using HTML CSS JavaScript —
Create a new project folder and organize it with the necessary files. You’ll need an HTML file (index.html)
, a CSS file (style.css)
, and a JavaScript file (script.js)
.
Structure the HTML:
Open your index.html file and structure the basic layout of your project. This includes defining the HTML elements for the text output, control settings, and code display.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Text Shadow Generator</title> </head> <body> <h1>Text Shadow Generator</h1> <div class="container"> <div id="output">Hello World</div> <div class="settings"> <label for="x-axis"> offset-x: <input type="range" name="x-axis" id="x-axis" min="0" max="100" value="10" step="5" /></label> <label for="y-axis"> offset-y: <input type="range" name="y-axis" id="y-axis" min="0" max="100" value="10" step="5" /></label> <label for="blur"> blur: <input type="range" name="blur" id="blur" min="0" max="50" step="5" value="10" /></label> <label for="color"> color: <input type="color" name="color" id="color" /></label> </div> </div> <div id="code"> <p>text-shadow:</p> 10px 10px 10px #000000; </div> </body> </html>
Style Your Project:
Utilize the style.css file to enhance the visual appeal of your Text Shadow Generator. Customize fonts, colors, and layouts to create an aesthetically pleasing interface.
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@400;500;600;800&display=swap"); * { margin: 0; padding: 0; } body { height: 100vh; display: flex; flex-direction: column; align-items: center; justify-content: center; font-family: "poppins"; background: linear-gradient(to right, #0f2027, #203a43, #2c5364); /* W3C, IE 10+/ Edge, Firefox 16+, Chrome 26+, Opera 12+, Safari 7+ */ } h1 { font-family: "poppins"; font-size: 4rem; margin-bottom: 50px; text-shadow: 5px 5px 30px #000000; } .container { width: 800px; display: flex; gap: 5px; } #output, .settings { width: 50%; border: 2px solid black; height: 200px; border-radius: 10px; background-color: rgba(255, 255, 255, 0.5); } #output { display: flex; align-items: center; justify-content: center; font-size: 50px; font-family: "poppins"; text-shadow: 10px 10px 10px black; } .settings { display: flex; flex-direction: column; align-items: center; justify-content: space-evenly; text-transform: capitalize; } .settings label { display: flex; justify-content: space-between; align-items: center; width: 80%; font-size: 30px; } .settings label input { height: 40px; width: 50%; } #code { display: flex; gap: 5px; color: orangered; background-color: black; text-align: center; margin-top: 20px; padding: 10px 20px; font-family: "poppins"; font-size: 20px; width: 450px; border-radius: 10px; color: lightgreen; } #code p { color: dodgerblue; } @media screen and (max-width: 900px) { .container { align-items: center; flex-direction: column; } }
Implement JavaScript Functionality:
Open script.js and implement the dynamic functionality. Utilize event listeners for input changes to update the text shadow in real-time. The code provided ensures a seamless user experience.
let x = document.getElementById("x-axis").value; let y = document.getElementById("y-axis").value; let blur = document.getElementById("blur").value; let color = document.getElementById("color").value; document.getElementById("x-axis").addEventListener("input", (e) => { x = e.target.value; document.getElementById( "output" ).style.textShadow = `${x}px ${y}px ${blur}px ${color}`; updateCode(); }); document.getElementById("y-axis").addEventListener("input", (e) => { y = e.target.value; document.getElementById( "output" ).style.textShadow = `${x}px ${y}px ${blur}px ${color}`; updateCode(); }); document.getElementById("blur").addEventListener("input", (e) => { blur = e.target.value; document.getElementById( "output" ).style.textShadow = `${x}px ${y}px ${blur}px ${color}`; updateCode(); }); document.getElementById("color").addEventListener("input", (e) => { color = e.target.value; document.getElementById( "output" ).style.textShadow = `${x}px ${y}px ${blur}px ${color}`; updateCode(); }); function updateCode() { document.getElementById( "code" ).innerHTML = `<p>text-shadow:</p> ${x}px ${y}px ${blur}px ${color};`; }
Conclusion:
Congratulations! You’ve successfully crafted a Text Shadow Generator using JavaScript, HTML, and CSS. This project not only reinforces your coding expertise but also provides a practical tool for experimenting with text shadow effects.
As you explore the code, feel free to modify parameters such as offset, blur, and color to see how they influence the text shadow. Remember, coding is an exploration – enjoy the process of building and refining your projects.
For your convenience, the total source code of this “Text Shadow Generator using JavaScript” project instructional practice is accessible for download by clicking the Download Code button.
Note: Keep in mind that the way to dominate coding is practice. To enhance your skills in JavaScript, HTML & CSS, we recommend recreating other useful website elements such as Custom Button, Reviews Card, Contact Page, Navigation, Login Forms, etc.
More Categories:
Blog • Cards • Fun Project • Game • Hamburger Menu • Login / Signup • Navbar • Testimony / Reviews • To-Do List